If you have a few routine SQL statements to execute, you can save them in a *.sql file and login to MySQL console to issue command source. Alternative, you can run these commands using PHP function.
Save the following database credentials in a separate PHP file.
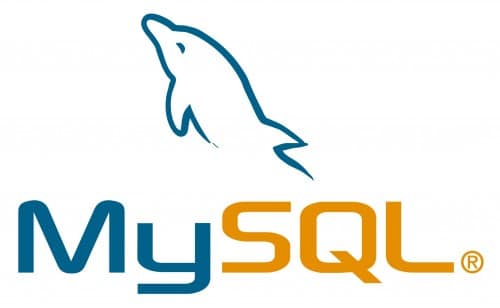
MySQL
1 2 3 4 5 | // conn.php define('DB_HOST', 'helloacm.com'); define('DB_USER', 'DB_USER'; define('DB_PASSWORD', 'DB_PASSWORD'); define('DB_NAME', 'DB_NAME'); |
// conn.php define('DB_HOST', 'helloacm.com'); define('DB_USER', 'DB_USER'; define('DB_PASSWORD', 'DB_PASSWORD'); define('DB_NAME', 'DB_NAME');
Then this does the job by reading the SQL script file and execute them each one by one, and line by line only. You would then need to invoke the function ExecSqlFile($filename.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | require('conn.php'); mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); mysql_query("SET NAMES utf8"); mysql_select_db(DB_NAME); function ExecSqlFile($filename) { if (!file_exists($filename)) { return false; } $querys = explode("\n", file_get_contents($filename)); foreach ($querys as $q) { $q = trim($q); if (strlen($q)) { mysql_query($q) or die(mysql_error()); } } return true; } |
require('conn.php'); mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); mysql_query("SET NAMES utf8"); mysql_select_db(DB_NAME); function ExecSqlFile($filename) { if (!file_exists($filename)) { return false; } $querys = explode("\n", file_get_contents($filename)); foreach ($querys as $q) { $q = trim($q); if (strlen($q)) { mysql_query($q) or die(mysql_error()); } } return true; }
You can save these two files and put it on crontab job, so that it can be executed on a scheduled basis.
–EOF (The Ultimate Computing & Technology Blog) —
GD Star Rating
loading...
274 wordsloading...
Last Post: C++ Coding Exercise - xxd - make hex dump on Windows
Next Post: C++ Coding Exercise - Parallel For - Monte Carlo PI Calculation
define(‘DB_USER’, ‘DB_USER’;
->
define(‘DB_USER’, ‘DB_USER’);