C# 6.0 has many handy new features and the string interpolation is one of my favorites. Let’s look at the simple code that uses the string.format function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | using System; namespace ConsoleApplication5 { class Program { static void Main(string[] args) { int v = 1; Console.WriteLine(string.Format("v = {0}", v)); Console.ReadLine(); } } } |
using System; namespace ConsoleApplication5 { class Program { static void Main(string[] args) { int v = 1; Console.WriteLine(string.Format("v = {0}", v)); Console.ReadLine(); } } }
The Resharper greys out the string.Format and says it is redundant call. It even offers a quick button to make it shorter/concise (the yellow bulb icon)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | using System; namespace ConsoleApplication5 { class Program { static void Main(string[] args) { int v = 1; Console.WriteLine($"v = {v}"); Console.ReadLine(); } } } |
using System; namespace ConsoleApplication5 { class Program { static void Main(string[] args) { int v = 1; Console.WriteLine($"v = {v}"); Console.ReadLine(); } } }
You see, the string interpolation puts a $ (dollar) sign before the string literal (similar to @ for not escaping strings). And the variables are directly put into the curly braces, so you don’t have to count the order of parameters.
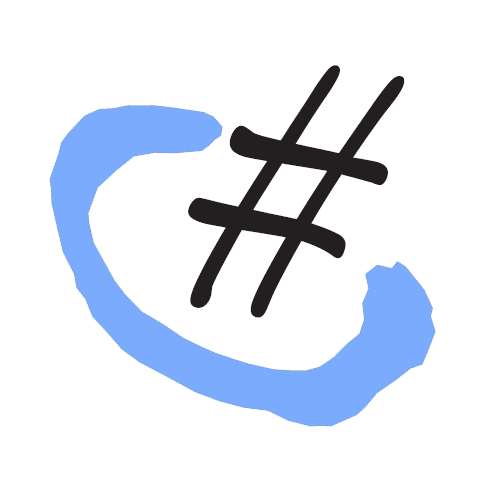
csharp
–EOF (The Ultimate Computing & Technology Blog) —
GD Star Rating
loading...
222 wordsloading...
Last Post: Keyboard Review: Razer BlackWidow Mechanical 87 Keys - Razer BlackWidow 2014 Tournament Edition Essential Mechanical USB Keyboard
Next Post: How to Generate the QR images in Batch using Google-Drive Spread Sheet?