Suppose you have a few important web pages (URL) and you want to make sure that these URLs are accessible before any further functions testing. So you can unit test the connectivity of these URLs by using the API:
https://helloacm.com/api/can-visit/
This API takes a url parameter which can be passed by GET or POST. And by using the PHPUnit, the famous and easiest PHP Unit Testing framework, you can test like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | <?php use PHPUnit\Framework\TestCase; class API_CanVisit_Tests extends TestCase { private $API = "https://helloacm.com/api/can-visit/"; public function URLProvider() { return [ ["https://happyukgo.com"], ["https://helloacm.com"], ["https://codingforspeed.com"], ["https://justyy.com"], ["https://weibomiaopai.com"], ["https://rot47.net"], ["https://uploadbeta.com"], ["https://isvbscriptdead.com"], ["https://helloacm.com"], ["https://steakovercooked.com"] ]; } /** * @dataProvider URLProvider */ public function test_url($url) { $data = file_get_contents("$this->API?url=$url"); $result = json_decode($data, true); $this->assertEquals(true, $result['result']); $this->assertEquals(200, $result['code']); } /** * @dataProvider URLProvider */ public function test_url_via_curl($url) { $data = shell_exec("curl -X POST $this->API -d \"url=$url\""); $result = json_decode($data, true); $this->true, $result['result']); $this->assertEquals(200, $result['code']); } } |
<?php use PHPUnit\Framework\TestCase; class API_CanVisit_Tests extends TestCase { private $API = "https://helloacm.com/api/can-visit/"; public function URLProvider() { return [ ["https://happyukgo.com"], ["https://helloacm.com"], ["https://codingforspeed.com"], ["https://justyy.com"], ["https://weibomiaopai.com"], ["https://rot47.net"], ["https://uploadbeta.com"], ["https://isvbscriptdead.com"], ["https://helloacm.com"], ["https://steakovercooked.com"] ]; } /** * @dataProvider URLProvider */ public function test_url($url) { $data = file_get_contents("$this->API?url=$url"); $result = json_decode($data, true); $this->assertEquals(true, $result['result']); $this->assertEquals(200, $result['code']); } /** * @dataProvider URLProvider */ public function test_url_via_curl($url) { $data = shell_exec("curl -X POST $this->API -d \"url=$url\""); $result = json_decode($data, true); $this->true, $result['result']); $this->assertEquals(200, $result['code']); } }
The API can be called using file_get_contents or the curl command (which can be shell_exec). The @dataProvider provides the data inputs to the test, so you can easily add more URLs. To run the unit tests, simply run phpunit given the following phpunit.xml in the same folder:
1 2 3 4 5 6 | <?xml version="1.0" encoding="utf-8" ?> <phpunit> <testsuite name='API Tests'> <directory suffix='_tests.php'>./</directory> </testsuite> </phpunit> |
<?xml version="1.0" encoding="utf-8" ?> <phpunit> <testsuite name='API Tests'> <directory suffix='_tests.php'>./</directory> </testsuite> </phpunit>
Alternatively, you can phpunit tests.php if you save above PHP Test code in tests.php. The example output by running PHPUnit is:
1 2 3 | Time: 9.34 seconds, Memory: 8.00MB OK (18 tests, 36 assertions) |
Time: 9.34 seconds, Memory: 8.00MB OK (18 tests, 36 assertions)
And here gives the example of running unit tests in the VPS Server.
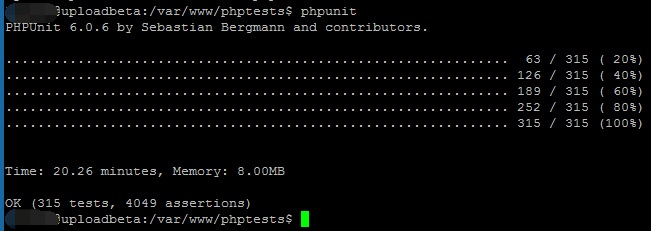
Run PHP Unit Tests
–EOF (The Ultimate Computing & Technology Blog) —
loading...
Last Post: PHP7 Shortens the Google Page Crawling Time
Next Post: Google Webmaster Reports Uncommon Downloads or Harmful Contents